Stacks Simplified
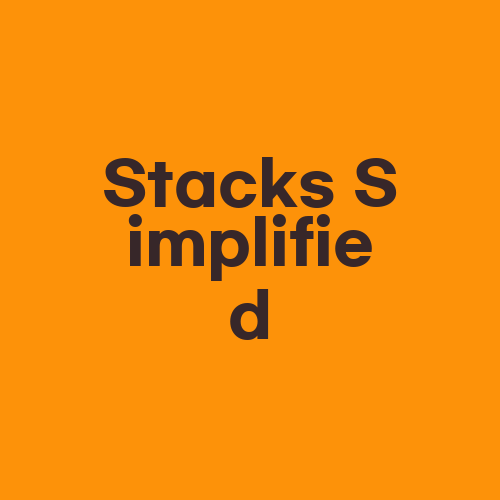
Stacks Simplified: A Beginner’s Guide to Learning Stacks
What are Stacks?
If you are a beginner interested in learning about computer science and data structures, then you may have come across the term “stacks”. In general, stacks are a collection of items that follows a specific pattern of access known as last in, first out (LIFO). This means that the item that was most recently added to the stack will be the first one to be removed.
Stacks are used in a wide array of applications, such as navigating web pages (via the browser history), in compilers for syntax analysis, and also in the implementation of method calls and recursion.
To better understand stacks, let’s take a look at a real-world example – a stack of plates. When you are adding plates to the stack, you place new plates on top of the previous one, i.e., the plate that was most recently added. When you are taking a plate away, you take it off the top of the stack, i.e., the one that was added most recently, and then work your way down.
Useful resources:
– https://en.wikipedia.org/wiki/Stack_(abstract_data_type)
– https://www.geeksforgeeks.org/stack-data-structure/
Stack Operations:
There are two fundamental operations performed on stacks, push and pop. Push adds a new element to the top of the stack, while pop removes the top element from the stack.
In addition to these two primary operations, stacks also support peek and isEmpty operations. The peek operation returns the top element without removing it, while the isEmpty operation returns true if the stack is empty.
Useful resources:
– https://www.tutorialspoint.com/data_structures_algorithms/stack_algorithm.htm
– https://www.programiz.com/dsa/stack
Implementing Stacks:
Stacks can be implemented using either arrays or linked lists. In the array implementation, a fixed-size array is used to store the stack. This implementation is simple and efficient, but it has a disadvantage in that the size must be fixed at the start and cannot be changed.
On the other hand, a linked list implementation allows for dynamic memory allocation, meaning that the size of the stack can change as needed.
When implementing stacks in programming languages like C/C++, Java, and Python, these languages provide built-in data structures for implementing stacks.
Useful resources:
– https://www.geeksforgeeks.org/stack-data-structure-introduction-program/
– https://www.educative.io/edpresso/array-implementation-of-a-stack-in-cpp
Common Applications of Stacks:
Stacks are used in a variety of applications, such as in the browser history of web pages, undo-redo functionality in software applications, and language compilers.
In web browsers, stacks are used to keep track of the web pages visited, allowing for easy navigation between pages.
Undo-redo functionality in software applications allows users to undo changes by moving back up the stack of changes or redo changes by moving down the stack.
In compilers, stacks are used in the implementation of language syntax analysis, helping to ensure that the code is written correctly.
Useful resources:
– https://www.studytonight.com/data-structures/stack-data-structure#
– http://www.cs.cornell.edu/courses/cs2112/2012sp/lectures/lec20/lec20-12sp.html
Frequently Asked Questions:
What is the difference between a stack and a queue?
Stacks and queues are both abstract data types that contain a collection of elements. However, stacks use a LIFO (last in, first out) order in which the last element added to the stack is the first to be removed, while queues use a FIFO (first in, first out) order in which the first element added to the queue is the first to be removed.
What is the maximum number of elements that can be added to a stack?
The maximum number of elements that can be added to a stack depends on the implementation method used. In the array implementation, the maximum number of elements is limited to the declared size of the array, while in the linked list implementation, there is no limit to the number of elements that can be added.
Can stacks only contain one type of element?
No, stacks can contain a mixture of element types, but it is more common for stacks to contain elements of a homogeneous type.
Conclusion
In conclusion, stacks are one of the most commonly used data structures in computer science. They provide a variety of applications, including navigating web pages, undo-redo functionality, and language compilers. If you are a beginner interested in learning about computer science and data structures, then understanding stacks is a good starting point.